Python: Validating Argparse Input with a List
- Brian
- Jun 3, 2021
- 2 min read
I've found that when attempting to validate the input for various scripts I run into common situations. I commonly have a list of acceptable values I want my script to accept, and reject everything else. Additionally, I found a nice method to use lists to validate user input.
My final solution can be seen below:
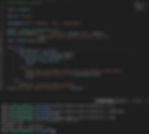
The way this code works by using the argparse Python module. The input is take via command line input and then compared against a list of values. If the value is present in the list of values, the new_var is then updated.
The new_var variable is declared with a value of "test". In the event that the variable is not updated, the user will be returned with a new_var variable that has the value of 'test'.
The acceptable_vars variable is a list that contained the variables which the CLI input needs to match.
Argparse was then used to create a command line switch which can be used as "-p" or "--practice". I used argparse in the following manor.
parser = argparse.ArgumentParser()
parser.add_argument("-p", "--practice", action="store", type=str,
help="Select which practice you want to go to")
args = parser.parse_args()
The input is then validated with a while loop.
First the presence of args.practice was tested with an if statement.
if the value was not present, the else clause printed out a warning letting the user know no --practice switch was provided.
Next, the args.practice was run through the lower method which converted the string value of args.practice to lower case. This made the practice command line switch case-insensitive.
The next test was an if statement determining if the value of args.practice after being run through the lower module was present in the list of predefined values.
if the value was present - the new_var variable would be updated with the value of test_new_var. Remember that variable is just holding args.practice.lower()
If the value was not present, the user was returned with a warning that the entry did not match an option in the list.
while True:
if args.practice is not None:
test_new_var = args.practice.lower()
if test_new_var in acceptable_vars:
new_var = test_new_var
break
else:
print("Your entry didn't match one of the options in the
list")
print("The options are:\n" + str(acceptable_vars))
print()
break
else:
print("No --practice value provided")
Here is me testing the code:
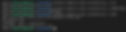
It can be seen that when 'bjj' was entered, the variable updated without issue since the value was in the list. It can also be seen that when WRESTLING was entered, it converted the string to lower and updated the new_var variable. When 'WoW was entered, I was returned with a warning that my selection was not in the list, the list of valid options, and an non-updated new_var variable holding a default value of 'test'.

Thanks for reading!
Read more about argparse here: https://docs.python.org/3/howto/argparse.html
Much of this solution was inspired from: https://stackoverflow.com/questions/33534751/checking-user-input-against-list-python-3-x